Wednesday, April 29, 2009
Firemint reveals sales figures for Flight Control
It's cool to get a peek into sales of a top rated app. One interesting note is that about 80% of their sales were to English speaking countries (the US, Great Britain, Canada and Australia), and considering the app isn't localized, that's 20% of their sales to non-English speaking countries. I wonder how localizing the app would change those numbers?
Monday, April 27, 2009
Love, Hate, C++ and Complexity
I learned and used C before learning C++. This was both beneficial and detrimental. C is sometimes called "high level assembly language" because statements and expressions in C map very closely to the generated machine code; the C compiler doesn't perform a lot of magical code generation for you. This engenders a "WYSIWYG" mindset in C programmers. When you see an expression like
x + y
, you know that the compiler is going to MOV
the contents of x
into a register, MOV
the contents of y
into another and ADD
them.C++ started off as "C with Classes". At its core, C++ classes are basically C
struct
s that act as namespaces for related functions. And there's a little syntactic sugar to hide the struct
pointer (this
) in these member functions and to perform initialization and clean up automatically. This doesn't seem like much, but when you're working with large code bases, going from:person_t *person = malloc(sizeof(person_t)); init_person(person, "Brian", "Griffin"); do_something_with_person(person); save_person(person, db); clean_up_person(person); free(person);to something a little cleaner and less repetitive:
Person *person = new Person("Brian", "Griffin"); person->doSomething(); person->saveIn(db); delete person;makes a big difference when repeated over and over.
Code for any significant system is written once but read many times. Anything that aids readability and organization pays off in the long run. By encouraging programmers to define their data structures and related functions together in a class instead of free form, C++ brought a level of organization to large code bases that was often not present in C. And because using classes and methods is more succinct than plain
struct
s and functions, readability of existing code increases. Not that you can't make a mess with C++, but if you're trying not to, there are some natural paths to organization in C++ that help.However, Bjarne Stroustrup is a computer language geek and sometimes it seems like he and the ANSI C++ committee never met a language feature they didn't like. Like the hydra, you'd master one new C++ feature and two new ones would sprout up in its place. Multiple inheritance, function overloading, operator overloading, templates, the STL, run time type information, exceptions, placement new, virtual inheritance, partial specialization, implicit conversions, inline functions, access specifiers, namespaces -- i'm sure I'm forgetting at least a dozen important concepts here.
I was drawn to C++ by the pain of writing the same boilerplate in C over and over. For instance, most moderate to large sized C programs use a linked list or two, but C doesn't give you good tools to encapsulate a linked list in a clean, compact, typesafe and reusable way. C++ does. There's even a perfectly cromulent linked list class in the C++ standard library. Moving from C to C++ was originally like moving from a bicycle to a motorcycle: it felt powerful and could take you places further and faster than you'd ever been before. But it was also a little scary. You're speeding along open and exposed, the wind buffeting your helmet and you know you can send yourself sliding down the asphalt if you're not careful.
I went through this love-hate cycle. I'd get disgusted with the limitations of C and turn to C++. It was always empowering at first -- classes, constructors, destructors, RAII, smart pointers,
string
s, vector
s, iterators. Then the dark underside of C++ would emerge. The impenetrable error messages, the mangled function names and oh my science, those paragraph-long template errors. I never had the misfortune of using Cfront, but those early versions of Borland and Microsoft C++ weren't very kind either. I'd lose hours, sometimes days trying to fix some bizarre syntax error. That was hard, but in the long run it drove me to acquire a deeper knowledge of C++. Once I understood what the little man behind the curtain was doing, things became a lot clearer.The more insidious side of C++ is also one of its attractions. With all the tools that it gives you, there are often many ways to approach a problem. Should I make something a
struct
or a class
? Should this data member be private
or protected
? Should I write a copy constructor for this class? Should I use inheritance, composition or templates to build my family of objects? If an exception is thrown in the middle of this constructor, will everything unwind properly? I declared a const
instance of this class, but I still need to change some of its internal data -- should I just make the data members mutable
or is this a code smell? Should I make this class a friend
of this other class, or am I really making a mess here? It's chock full of fun and interesting things to ponder, but I was often finding my progress grinding to a snail's pace as I struggled with all the choices that C++ gave me. Eventually I'd throw up my hands in disgust and go back to the happy world of C. Boilerplate be damned, at least I know what everything is doing and there's really not that many ways to do something. You write
struct
s and functions that act on them. About the most contentious issue is whether to create constants using #define
or enum
. In many ways, C is far more productive. No classes, no exceptions, no access specifiers, no templates, no overloading. You just crank out code. I'll give C++ credit here though -- it made me a better C programmer. My C code today is cleaner and better organized and about as "object oriented" as you can make C; C++ taught me that.After a while I'd tire of writing yet another linked list and revisit the exciting world of C++. This love-hate cycle eventually ended and I made my peace with both C and C++. I acquired a pretty expert knowledge of C++ and it's an old friend now. Mangled names no longer bother me and those template compiler error messages no longer daunt. It hasn't hurt that the spec was completed a decade ago in 1998 and the last revision was in 2005 with TR1. That's a lifetime in computer years.
My peaceful repose is about to end. The next major version of C++ is close to finalization. I had the good fortune of seeing Bjarne himself talk about it a couple of years ago at Google, but in the hour and a half he talked, he barely scratched the surface. Wikipedia has an extensive article on the new version. And recently, Stephan T Lavavej of Microsoft's Visual C++ team has written a series of articles on the Visual C++ Team Blog covering some of the new features in detail. Microsoft is adding support for many of the upcoming features into the next version of Visual C++, slated to be part of Visual Studio 2010.
In his first part, Stephan covers the new syntax for defining anonymous functions in expressions (known as lamdas, lambda expressions or lambda functions), type inference in variable declarations with the
auto
keyword and static_assert
for making compile-time assertions.Stephan's second part covers rvalue references which enable move semantics and perfect forwarding. Move semantics enables you to optimize construction and assignment from temporary objects to minimize memory allocation and copying. Perfect forwarding allows you to write template functions that preserve the
const
and lvalue/rvalue quality of their arguments, which is important for writing template based wrappers. The most recent part covers the new
decltype
keyword, which is used in template function declarations to specify return types that are deduced from function arguments.This is all heady stuff, but if you use C++, it's worth spending some time going through Stefan's examples, particularly in part 2. And be sure to take a peek at the Wikipedia article to get a sense of just how much new stuff will be a part of the upcoming standard. It'll be like a whole new language:
std::initializer_list
and uniform initialization, a new for
loop syntax, return types after the parameter list, concepts, nullptr
, enum class
es, template typedef
s, variadic templates, Unicode string literals, long long int
, std::unorded_map
(hash table), std::regex
(regular expressions) and more.As an armchair computer language geek and closet masochist, I'm looking forward to some fun-filled evenings parsing bizarre new compiler errors. As a working programmer and entrepreneur, I'm glad that I'm currently doing most of my coding in Objective-C and Python.
(11:30 AM: Fixed mistake in example code.)
Friday, April 24, 2009
Apple's App Store: One Billion Downloads
As of today one billion apps have been download from the Apple's App Store which has only been online for about 10 months. Roughly 100,000,000 apps per month or 3.3 million apps per day were being downloaded; that's just mind-boggling.
On top of the run-away success of App Store, Apple announced their financial results for its fiscal 2009 second quarter ending March 28, 2009 with the best march quarter revenue and earnings in apple history.
“We are extremely pleased to report the best non-holiday quarter revenue and earnings in our history...”
- Peter Oppenheimer, Apple’s CFO
So congratulations again Apple and thanks a billion!
App Store: And the winners are...
The other two winners are Apple and Bump Technologies. Apple for it's one billionth download showing to the world that design is everything and content is king. Bump Technologies for having their free contact sharing application Bump be the billionth download. Bump was released less than a month ago on March 27, 2009. Now if only it were a .99 cent app they would have seen a bump in their revenue. But press is press and good press is great press.
iPhone Friday - April 24, 2009
Monday, April 20, 2009
Apple's App Store: setting the bar for online app markets
Also, as I stated in a previous blog entry, even though Apple's App Store has only been available for about 10 months, App Store is about to hit 1 billion downloads currently at 986,084,397 downloads. Read the blog entry to learn how you can enter for free for a chance to win during their 1 billion downloads sweepstakes.
Friday, April 17, 2009
iPhone Friday - April 17, 2009
Space is big. Really big. You just won't believe how vastly hugely mind-bogglingly big it is. I mean, you may think it's a long way down the road to the chemist, but that's just peanuts to space.The eleven images in todays iPhone Friday are only a few from Saturn, Jupiter, their moons and or rings. I'll post more wallpapers from Photojournal later as I have many that I made for myself over the years. Enjoy.
- Douglas Noel Adams
Wednesday, April 15, 2009
Exploring the Objective-C Runtime
Tuesday, April 14, 2009
Google Chart API is Cool
Since I'm writing a sales application, I needed a way to produce charts. I've played around with the
<canvas>
tag on a previous project, but really wanted something higher level. My boyfriend Jerry suggested I look at the Google Chart API. It's wonderfully simple: you build a URL like http://chart.apis.google.com/chart?cht=p3&chd=t:60,40&chs=250x100&chl=Hello|World
and use it in an <img>
tag. Google renders up your chart as a PNG and sends it back to your browser, like this:My only criticism is the brevity of the URL parameters (
cht
is chart type, chxl
is axis labels, etc.) and the various ways of delimiting multivalued parameters (data takes a comma delimited list, labels are pipe '|' delimited) can be trying to work with at times. Still, after a couple of hours of modest twiddling, I had a couple of Python functions to build URLs for a bar chart and pie chart, respectively and my sales app has two respectable looking charts on the home page. Google Chart API is definately worth taking a look at.
Monday, April 13, 2009
App Store about to hit a billion download - offers free entry to win
comScore's Top 25 Apps
Most interesting was the heavy concentration of games in the list -- 12 of the 25 are in the games category. Facebook, MySpace and AOL Instant Messenger apps also make the top 25 cut. If you consider social networking apps to be part of a broad entertainment category that includes music and games, the only serious apps on the list are Google Earth and Flashlight (and Google Earth is pretty much entertainment too).
Friday, April 10, 2009
iPhone Friday - April 10, 2009
Hey there! It's Friday. The weekend is upon us. With it come good times, relaxing afternoons and more iPhone Wallpapers. As today is the tenth of April, I am presenting ten wallpapers of Chinese artwork and four from my Photography collections. First the Chinese artwork.
These are from the DoverPictura series of book offered from Dover Publications. I with the black and white EPS files, using them as a stencil then coloring them in with various gradients. I personally like doing four first, the seasons, as an opening exercise. Bellow there are six total; two of a deer and four of a medallion.
The following four from the Photography collection were taken in San Francisco's beautiful City Hall at a friends marriage ceremony. There was a lot going on that afternoon as you can see in the images bellow.
Okay, that's if for today. See you next week and have a great weekend!
Thursday, April 9, 2009
Tips on Marketing Your Startup
While I would quibble with some of his points, I definitely agree with many of them, and the overall theme of his post is to get engaged in stuff, get out there and listen to what people are saying and add your 0.02 USD -- good advice for us techies who are stuck all day with our nose to the monitor (LOLcats doesn't count here ;-).
Wednesday, April 8, 2009
Review: The first three lectures from Stanford's iPhone Application Development
I subscribed to the podcast and sync it with my iPhone. Having the materials with me allows me to study on the go. It's a little bit of a mindgasm. I am quite pleased with the depth and quality of the information presented by Evan Doll and Alan Cannistraro thus far.
In addition, Stanford's website has a course information page with handouts, assignments, sample code and walkthroughs are posted. This makes the experience just that much more immersive and rich. It's like they said, "this is cool but how can we make it better." And they did just that. Thank you guys. I even enjoyed being "Rick-rolled" in the first lecture. (^_^)
Announcing Animals?
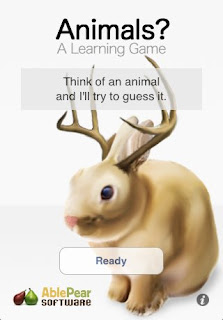
Animals? is a game of questions and answers where you think of an animal and Animals? tries to guess it. When Animals? guesses wrong, you teach it a new animal and a new question to ask.
Animals? was inspired by a very old computer game written in BASIC called Animal, originally developed by Arthur Luehrmann at Dartmouth College. I first came across Animal in the early 80's -- it was one of the sample programs that was included with Applesoft BASIC that shipped with every Apple II computer at the time.
We hope you have as much fun playing Animals? as Kevin and I had making it!
Click here to see our complete list of apps.
Tuesday, April 7, 2009
Speed of iPhone OS Upgrades
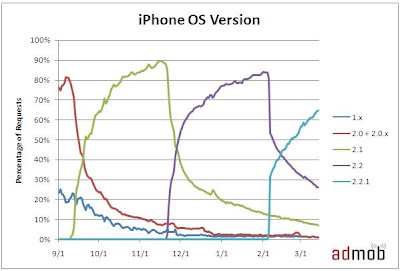
Friday, April 3, 2009
iPhone Friday - April 3, 2009
Good morning and happy Friday. iPhone Friday.
First, a not-so-random shout-out to France, Greece, Japan, Switzerland, the United Kingdom and the United States. Thank you.
Second, the meat of this iPhone Friday blog entry - wallpapers. Last week we presented slides (and a video) on how-to save images from within the Safari browser on an Apple iPhone or iPod Touch. We quickly followed up with a set of four wallpapers from the Innersquare collection. Today we present to you four from the Innersquare collection.
Additionally, I’m including a set of four from our nature photography collection. Enjoy.
Last, if you have any comments or requests please post them. Participate.
See you next Friday. (^_^)